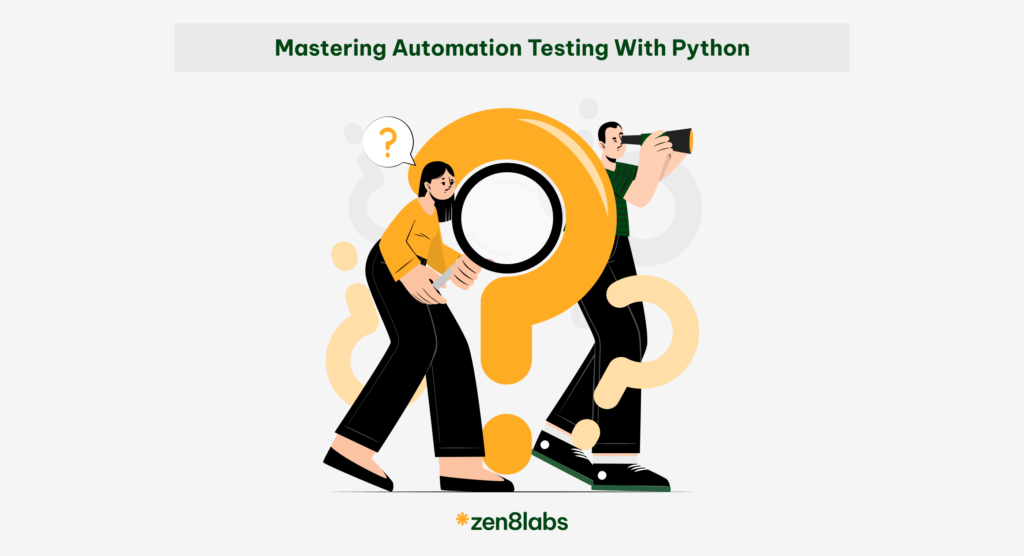
Automation testing has transformed the way software development teams of zen8labs ensure the reliability and functionality of their applications. As modern software becomes more complex, manual testing alone isn’t scalable. Automation testing fills this gap by allowing repeated execution of predefined test cases, ensuring that applications meet quality standards efficiently.
Python has emerged as a popular language for automation testing due to its readability, flexibility, and the availability of powerful libraries such as Selenium, PyTest, and Requests. This blog will guide you through the entire landscape of automation testing with Python, from the basics to advanced techniques. Whether you’re a beginner or an experienced tester, this guide will help you understand how Python can streamline your testing processes.
1. Introduction to automation testing
What is automation testing?
Automation testing refers to using software tools and scripts to automatically execute test cases and verify results against expected outcomes. It eliminates the need for manual intervention in repetitive tasks and allows tests to be performed at a scale.
Automation testing is crucial in modern software development. As projects grow and software is continuously integrated, the need to assure functionality without human error becomes essential. Automated tests are typically used to validate various aspects of software such as functionality, performance, security, and more.
Benefits of automation testing
- Efficiency: Automated tests run faster than manual tests and can be executed 24/7.
- Scalability: You can run tests on multiple platforms, devices, and environments simultaneously.
- Reusability: Once written, test scripts can be reused in different projects and adapted with minimal effort.
- Consistency: Automated tests produce consistent results, reducing the chance of human error.
- Cost savings: Although initial setup may be expensive, the long-term savings in time and resources make it worth the investment.
- Faster feedback: Automated tests provide instant feedback on the quality of the software, enabling faster bug identification and resolution.
Why choose Python for automation testing?
Python is an excellent choice for automation testing for several reasons such as:
- Readability: Python’s clean and simple syntax makes it easy to read and write test scripts.
- Extensive libraries: Python has a vast ecosystem of libraries like Selenium for browser automation, PyTest for unit testing, and Requests for API testing.
- Cross-platform: Python runs on all major operating systems, making it ideal for cross-platform testing.
- Community support: With Python being one of the most popular languages, there’s an abundance of tutorials, documentation, and community support available.
2. Setting up Python for automation testing
Installing Python
To get started with Python for automation testing, you first need to install Python. Visit the (official Python website – https://www.python.org/downloads/) and download the latest version. Once installed, verify the installation by running the following command in your terminal:

Setting up a virtual environment
A virtual environment helps you isolate your project’s dependencies from other Python projects on your system. Here’s how to set up a virtual environment:
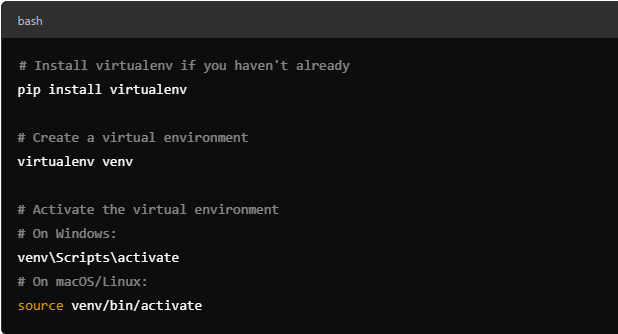
Installing essential libraries
For automation testing, you’ll need several libraries. Let’s install some of the key ones:
1. Selenium for browser automation:

2. PyTest for unit and functional testing:

3. Requests for API testing:

3. Web automation with Selenium
Selenium is one of the most popular tools for automating web browsers. With Selenium, testers at zen8labs can simulate user interactions such as clicking buttons, filling out forms, navigating between pages, and verifying page content.
Installing web drivers
Selenium works by communicating with a browser through a driver. For Chrome, you’ll need to download [ChromeDriver](https://googlechromelabs.github.io/chrome-for-testing/) and ensure its accessible from your system’s PATH.
A simple Selenium test script
Here’s an example of how you can use Selenium to automate a Google search:
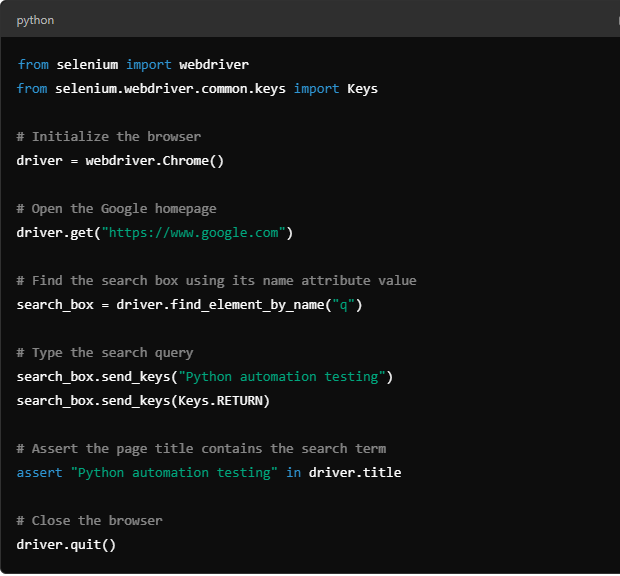
In this script:
- We open Chrome, navigate to Google, perform a search, verify the page title, and close the browser.
- Selenium automates every interaction with the web page just like a user would.
Handling web elements
Selenium allows zen8labs’ testers to be able to execute tests that interact with various web elements including buttons, links, forms, and dropdowns. You can locate elements using their ID, name, class, CSS selector, or XPath.
Example of locating and clicking a button:

Waiting for elements
Web applications often have dynamic content that takes time to load. Using sleep()
to wait is inefficient. Instead, Selenium provides explicit waits:
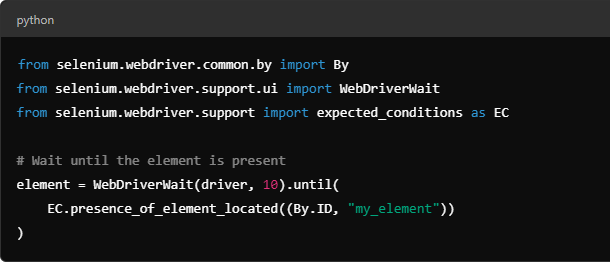
Headless browser testing
Sometimes, you might want to run tests without opening a browser window, which is useful in CI/CD environments. Selenium supports headless mode:
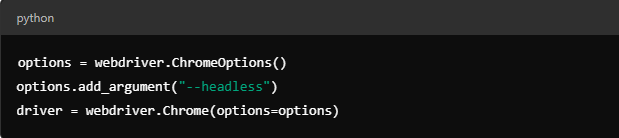
4. Unit testing with PyTest
PyTest is a powerful and easy-to-use framework for writing and running Python tests. It’s perfect for both unit and functional testing.
Writing your first PyTest test
Here’s an example of a basic test with PyTest:
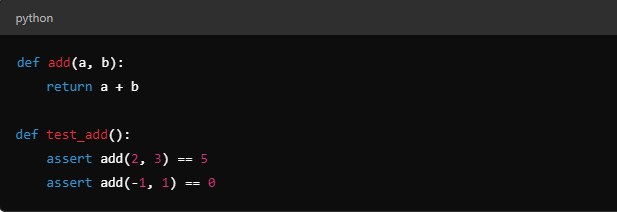
Save this as test_example.py
and run it with PyTest:

Grouping tests
You can group related tests using classes or naming conventions:
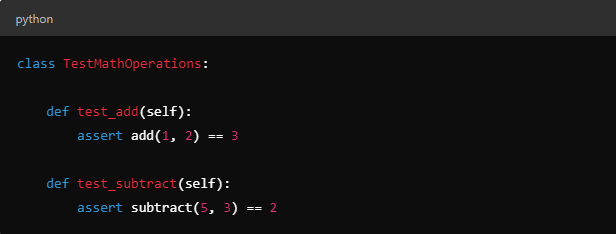
Fixtures
Fixtures allow you to define setup and teardown methods for tests. For example, initializing a database connection or opening a browser:
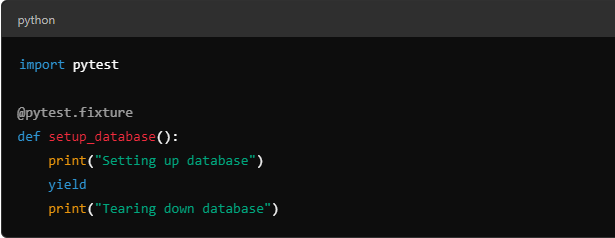
You can then use the fixture in your tests:

5. API testing with Python
Automation testing isn’t just limited to web applications, it can also use Python to test APIs. The Requests library is widely used for sending HTTP requests and validating responses.
Sending API requests with requests
Here’s an example of sending a GET request and checking the response:
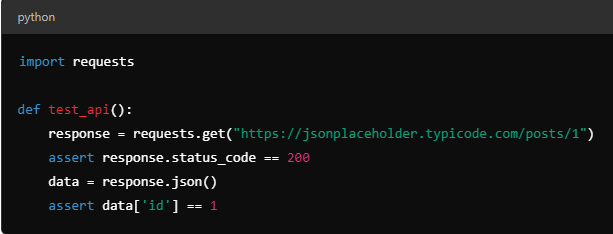
Testing with authentication
For APIs that require authentication, you can pass credentials with your request:
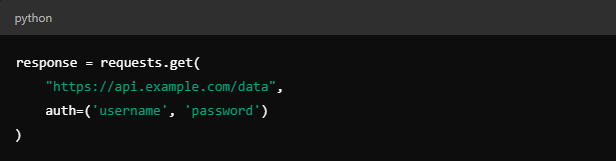
Automating API testing
You can combine Requests with PyTest to automate API testing:
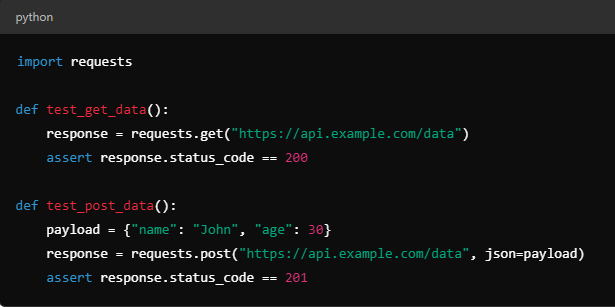
6. Integrating automation testing with CI/CD
Continuous Integration (CI) ensures that code changes are automatically tested when they’re pushed to a repository. Continuous Deployment (CD) extends this by automatically deploying code if all test passes. There are many popular CI/CD tools that can integrate well with Python automation tests like Jenkins, Travis CI, and GitLab CI.
Configuring Jenkins for automation testing
- Install Jenkins and configure it with your repository.
- Set up a job that runs your automation tests on every push.
- Add a shell command in Jenkins to run your tests:

Adding a Travis CI configuration
For projects hosted on GitHub, you can use Travis CI. Add a .travis.yml file to your repository:
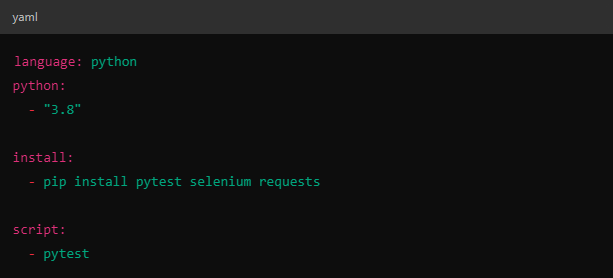
7. Advanced automation testing techniques
Data-driven testing
Data-driven testing allows you to run the same test with different sets of data. PyTest provides support for parameterized tests:
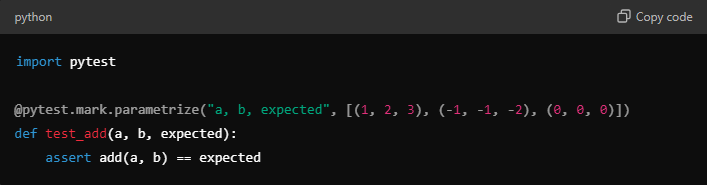
Parallel testing
Parallel testing involves running multiple test cases simultaneously to save time. PyTest provides the pytest-xdist plugin for this purpose:

Continuous testing with docker
You can use Docker to ensure that your tests run in isolated environments:
- Create a Dockerfile to set up your environment.
- Use docker-compose to run your tests in multiple environments simultaneously.
8. Best practices for automation testing
Effective automation testing necessitates a structured and maintainable approach to ensure the durability and reliability of test scripts as applications progress. Adhering to best practices minimizes technical debt, enhances test efficiency, and maintains the accuracy and relevance of test outcomes.
Maintainable code
- Modularize Tests: Break down tests into small, independent pieces.
- Reusable Functions: Create helper functions for repetitive tasks (e.g., logging in).
- Avoid Hardcoding: Use configuration files or environment variables to store sensitive data.
Regular maintenance
- Refactor Tests Regularly: As your application evolves, so should your tests.
- Monitor Test Performance: Slow tests can indicate inefficiencies in the code.
- Continuous Feedback: Ensure that test results are communicated to the development team quickly and efficiently.
Conclusion
Automation testing with Python provides a scalable, and efficient solution for ensuring the quality of modern software. Whether zen8labs’ testers automating web applications with Selenium, running unit tests with PyTest, or testing APIs with Requests, Python’s vast ecosystem of tools can simplify your workflow and improve the reliability of your applications. Accommodating these tests into your CI/CD pipelines and following best practices, you’ll be well on your way to building maintainable and scalable automation frameworks. There are a lot more helpful insights on our website, check them out!
Bich Nguyen – Quality Assurance Engineer