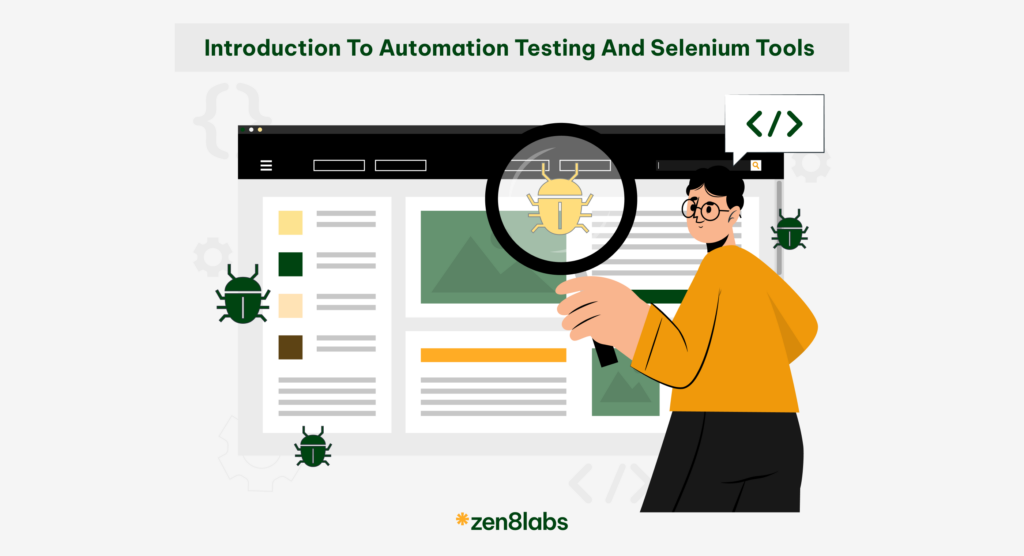
Automation testing has become an indispensable part of the information technology industry. The most common reason is that automation testing helps save costs and time in executing test cases. Additionally, it allows for the implementation of complex testing processes, eliminates potential manual testing errors, and produces consistent, reliable results. So, what is automation testing? Let’s explore automation testing and the Selenium tool.
What is automation testing?
Automation testing is the process of using tools and software to execute test scripts automatically. Instead of performing manual testing, testers write test scripts in the form of code, which are then executed by automation testing tools, to check the functionality and performance of the software application.
Advantages and disadvantages
Advantages
Some advantages of automation testing include:
- High reliability: Automation testing tools offer high stability because they operate based on predefined processes. Particularly, in cases involving many test cases, they help avoid human errors that may occur during manual testing.
- Repeatability: You can easily test software functionality and performance when it involves running the same script repeatedly. This helps testers handle repetitive tasks such as data entry, result verification, and clicking. This is often referred to as performance or load testing.
- Reusability: With automation testing, you can reuse test scripts across multiple versions of the application, even if the user interface changes. You can also test in various environments such as beta and production.
- High speed: Automation testing runs tests at least ten times faster than manual testing. It takes only about 30 seconds to execute a test automatically compared to 5 minutes for manual testing.
- Low cost: When applied correctly and for the right purposes, automation testing can save significant costs in both time and labor. Automation testing is much faster than manual testing. Additionally, the manpower needed to create and maintain scripts is minimal.
Disadvantages
In addition to the disadvantages mentioned above, automation testing still faces some limitations:
- Difficult to scale and maintain: Scaling the scope of automation testing is much harder compared to manual testing within the same project. This is because updating or modifying requirements involves a lot of tasks such as debugging, changing input data, and updating new code.
- Suitable for maintained projects with few system changes: System changes require adjustments to test cases, which can be costly and time-consuming in terms of editing auto test code.
- Low coverage: Due to the requirement for programming skills and difficulty in scaling, the coverage of automated testing, when viewed from the perspective of the entire project, tends to be lower.
- Time-consuming: The preparation phase for testing, such as writing test cases, can be time-consuming.
Getting started with automation testing
To begin with Automation Testing, you need to do the following:
- Choosing an appropriate testing tool: This is based on the project’s requirements and technology.
- Learn a programming language: Understand the basics of the programming language that the testing tool uses.
- Write test scripts: Develop detailed and clear test scripts.
- Implement and maintain testing: Run automated test scripts and update them as necessary.
Popular Automation Testing tools
There are several tools available to support automated testing, depending on the needs and programming languages used:
- Selenium: The most popular tool for testing web applications, supporting multiple programming
- languages such as Java, C#, Python.
- JUnit/TestNG: Unit testing libraries for Java.
- Cucumber: A tool supporting the writing of test scripts in the BDD (Behavior Driven Development) style.
- JMeter: A tool for performance testing.
- Appium: An automation testing tool for mobile applications.
Below, we’ll briefly discuss Selenium and how to use Selenium with the Java programming language.
What is Selenium?
Selenium is an open-source toolset used for automating tasks on web browsers. Created by Jason Huggins in 2004, Selenium has evolved into one of the leading automated testing tools, supporting multiple programming languages and different browsers.
Components of Selenium
Selenium consists of four main components:
- Selenium IDE: A handy tool available on Firefox and Chrome that allows recording and playback of interactions with the browser.
- Selenium WebDriver: The core component that enables direct interaction with the browser. WebDriver supports multiple programming languages, including Java, C#, Python, Ruby, and JavaScript.
- Selenium Grid: Enables parallel testing across multiple machines and different browsers.
- Selenium RC (Remote Control): An older tool, now less commonly used compared to WebDriver.
Why use Selenium with Java?
- Wide support: Java is one of the most popular programming languages and is widely supported in the Selenium community.
- High performance: Java is known for its high performance and powerful processing capabilities.
- Easy integration: Selenium easily integrates with test management and CI/CD tools such as Maven, Jenkins, and TestNG.
Installation of Selenium with Java
To get started with Selenium WebDriver and Java, you need to follow these steps:
- Install JDK: Download and install the Java Development Kit (JDK) from oracle.com.
- Set up the development environment: Use an IDE such as Eclipse or IntelliJ IDEA.
- Create a Maven project: Maven helps manage libraries and dependencies easily.
- Add dependencies for Selenium: Add Selenium dependencies to the pom.xml file.
Example of pom.xml:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>selenium-java-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
- Download WebDriver for the browser: For example, download ChromeDriver from chromedriver.chromium.org.
Write a simple test script with Java
Here is an example of a simple test script using Selenium WebDriver with Java:
A class containing a simple function to open a web page
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class OpenWeb {
public static WebDriver Open(String web) {
System.setProperty("webdriver.chrome.driver", "D:\\Setup File\\chromedriver.exe");
ChromeOptions options = new ChromeOptions();
options.addArguments("--incognito");
WebDriver driver = new ChromeDriver(options);
driver.get(web);
return driver;
}
}
A simple Class containing a function for registering and logging into a website:
WebElement login = driver.findElement(By.xpath("//*[@id=\"_desktop_user_info\"]/div/a/span"));
login.click();
Thread.sleep(500);
WebElement createaccount = driver.findElement(By.xpath("//*[@id=\"content\"]/div/a"));
createaccount.click();
WebElement gender= driver.findElement(By.xpath("//*[@id=\"customer-form\"]/section/div[1]/div[1]/label[1]/span/input"));
gender.click();
WebElement firstname= driver.findElement(By.name("firstname"));
firstname.sendKeys("Harry");
WebElement lastname= driver.findElement(By.name("lastname"));
lastname.sendKeys("Potter");
WebElement email= driver.findElement(By.name("email"));
email.sendKeys("potterh@gmail.com");
WebElement pwd= driver.findElement(By.name("password"));
pwd.sendKeys("Van23595");
WebElement show= driver.findElement(By.xpath("//*[@id=\"customer-form\"]/section/div[5]/div[1]/div/span/button"));
show.click();
WebElement bday= driver.findElement(By.name("birthday"));
bday.sendKeys("05/23/1995");
WebElement agree= driver.findElement(By.name("psgdpr"));
agree.click();
WebElement btgS= driver.findElement(By.xpath("//*[ @id=\"customer-form\"]/footer/button"));
btgS.click();
WebElement checkSuccess= driver.findElement(By.xpath("//*[@id=\"_desktop_user_info\"]/div/a[1]/i"));
if(checkSuccess.getText()!=null) {
System.out.println("Success");
driver.quit();
}else {
System.out.println("fail");
driver.quit();
}
}
/*
*
* Login
*
*
*/
public static void login() throws InterruptedException{
String web = "http://teststore.automationtesting.co.uk/";
driver= OpenWeb.Open(web);
Thread.sleep(1500);
WebElement signin = driver.findElement(By.xpath("//* [@id=\"_desktop_user_info\"]/div/a/span"));
signin.click();
Thread.sleep(500);
WebElement acc= driver.findElement(By.name("email"));
acc.sendKeys("potterh@gmail.com");
WebElement pwd= driver.findElement(By.name("password"));
pwd.sendKeys("1234567");
WebElement btglogin= driver.findElement(By.id("submit-login"));
btglogin.click();
}
}
Conclusion
Selenium is a powerful and versatile tool for automating tasks on web browsers effectively. Combined with Java, Selenium becomes extremely robust and effective to integrate into your automated testing workflow. Hopefully, this article has provided you with fundamental knowledge and a starting point for using Selenium with Java. In the next article, we will explore setting up the environment and requirements for Selenium Java. If this till seems like a lot of information to process, then reach out to zen8labs, let us help you.
Viet-Anh Nguyen, Quality Assurance Engineer