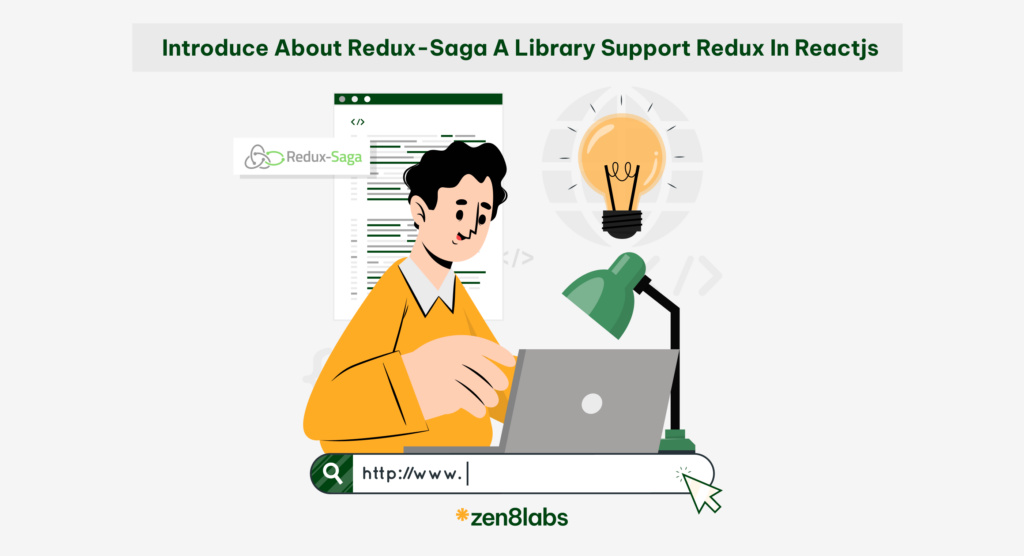
Introduction
For beginners exploring Redux, you’ll come across many tutorials using Redux Thunk or Redux Saga to manage async actions. However, experts often recommend using Redux Saga over Redux Thunk in large-scale projects. So, what is Redux Saga? How do you set it up? What are its advantages and disadvantages? Let zen8labs break down all these areas for you.
What is Redux Saga?
Redux Saga is a library that helps manage the side effects of an application – such as asynchronous tasks like data fetching and impure tasks like accessing the browser cache – making them easier to handle, more efficient to execute, easier to test, and better at handling errors.
Redux Saga is middleware for Redux (Redux is an open-source JavaScript library for managing application state, commonly used with libraries like React or Angular to build user interfaces). This Redux middleware can be started, paused, and canceled from the main application using standard Redux actions.
Redux Saga has access to the full state of the Redux application and can dispatch Redux actions.
Redux Saga uses an ES6 feature called Generators, which makes these asynchronous flows easy to read, write, and test. Using Redux Saga, these asynchronous flows look like your standard synchronous JavaScript code.
What is a generator function?
A generator function is a function that can pause its execution before completion and can resume at a later point. This feature of Redux Saga helps us handle tasks in a synchronous manner. The function will pause and wait for the async task to be completed before continuing its work.
Example: To understand this function better in Redux Saga, let’s look at a simple example below:
Example of a Redux Saga generator function
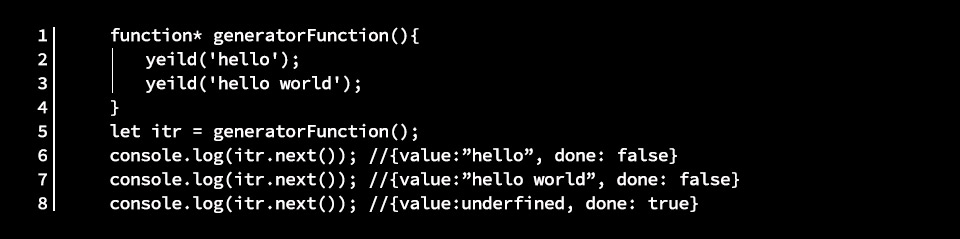
Calling a generator function does not execute it immediately; instead, Redux returns an iterator object. When we call the next method on the iterator object, the function body executes until it encounters the next “yield” keyword.
The next method returns an object with a “value” property that holds the returned result and a “done” property indicating whether the function has completed execution.
Basic terminology in Redux Saga
Redux Saga provides several methods called effects, as follows:
- Fork: Non-blocking call mechanism on a function
- Call: Calls a function. If Redux Saga returns a promise, it pauses the Saga until the promise is resolved
- Take: Pauses the application until an action is received
- Put: Dispatches an action
- takeEvery: Monitors changes in a specific action to call a Saga
- takeLatest: Executes a series of actions and returns the result of the last action
- Yield: Runs sequentially, continuing to the next action only after receiving a result
- Select: Runs a selector function to get data from the previous state
Installing Redux Saga
You can install Redux Saga in one of two ways:
$ npm install redux-saga
or
$ yarn add redux-saga
Additionally, you can use the UMD builds provided directly in the HTML script tag.
When to use Redux Saga
Using Redux Saga is beneficial when you want to perform an action without altering the application state.
Additionally, Redux Saga is advantageous in the following specific cases:
- Making HTTP requests to the server.
- Sending WebSocket events.
- Storing items in the browser cache or local storage.
How does Redux Saga work?
Redux is based on a flux architecture and supports unidirectional data flow. The data in the application cycles through the same loop repeatedly, making everything more predictable.
Advantages and disadvantages of Redux Saga
Using Redux Saga to manage application side effects offers many interesting new experiences for users. However, Redux Saga has its pros and cons, as detailed below:
Advantages
- Easily predictable application state: The state in Redux is predictable because reducers are pure functions. If you use multiple actions simultaneously, Redux Saga will return the same result. Additionally, the state of Redux Saga remains unchanged or modified predictably.
- Easy maintenance: Due to the predictable state and strict rules on the structure of a Redux application, anyone familiar with Redux can understand and work with it easily.
- Easy debugging: Redux Saga allows you to record behavior using available developer tools, making debugging easier.
- Available developer tools: Redux has developer tools that can be used in the browser to see what happens in the backend.
- Server-side rendering: Redux Saga supports server-side rendering by managing initial rendering. Redux sends the application state to the server in response to server requests.
Disadvantages
- Complex Redux Saga syntax.
- Many concepts to remember: Redux Saga has numerous concepts that users must keep in mind.
Comparing Redux Saga and Redux Thunk
In completing projects, Redux Saga and Redux Thunk are both valuable tools for software developers. But have you ever compared these two tools?
Let’s allow GrowUpWork to do that in this article!
Redux Thunk | Redux Saga |
Less code | Additional console logs |
Easier to understand compared to Redux Saga | Difficult to understand due to many concepts like generator functions and Redux Saga effects |
Hard to scale | Easier to scale compared to Redux Thunk |
Action creators might hold too much async logic | Action creators are always clean |
Hard to test | Relatively easy to test as all async logic is together |
Redux Saga monitors dispatched actions and triggers API calls or any other side effects written in the code. Saga operates like a separate thread or background process entirely responsible for executing your side effects or API calls.
This is entirely different from Redux Thunk. Redux Thunk uses callbacks, which can lead to difficult situations in some cases.
Conclusion
In summary, both Redux Thunk and Redux Saga are excellent tools. However, you need to choose the approach that best suits your project requirements. For medium to small projects, Redux Thunk may be more useful due to its simplicity.
However, for larger projects, Redux Thunk can cause problems as it is hard to scale if your side effects or asynchronous logic increase. This is where Redux Saga becomes very useful and powerful. It provides tools for concurrent effects, canceling side effects, and scaling effects on a larger basis. If that seems to challenging still, then reach out to us.
Thanh Tran, Software Engineer