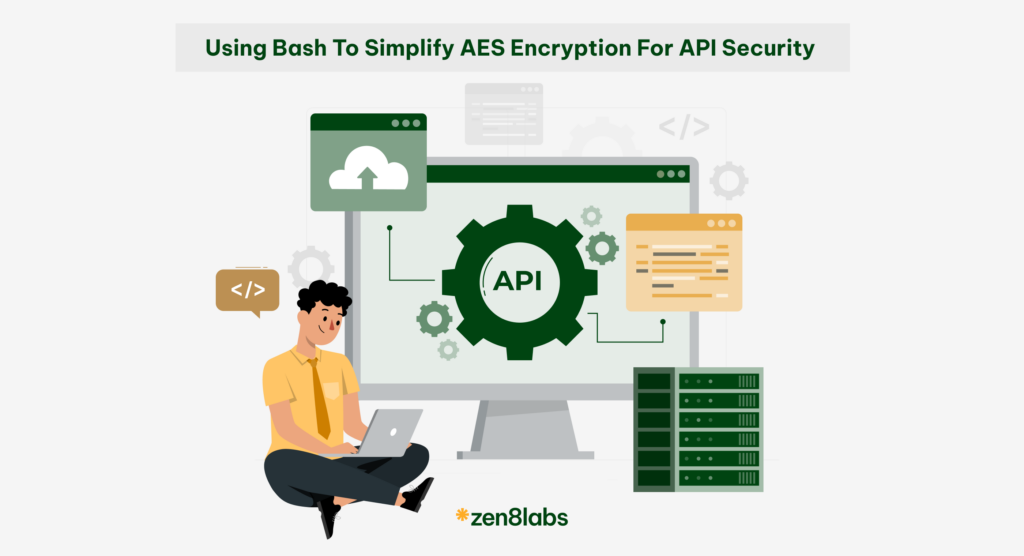
For zen8labs mobile developers, ensuring the security of data sent over APIs is important. The chosen encryption algorithm is AES since it is dependable and widely used. Encryption functions can be difficult to implement and time-consuming to check accurately. I’ve created a Bash script that makes AES encryption easier to speed up this step. I’ll guide you through writing and utilizing this script step-by-step in this blog.
Understanding AES encryption
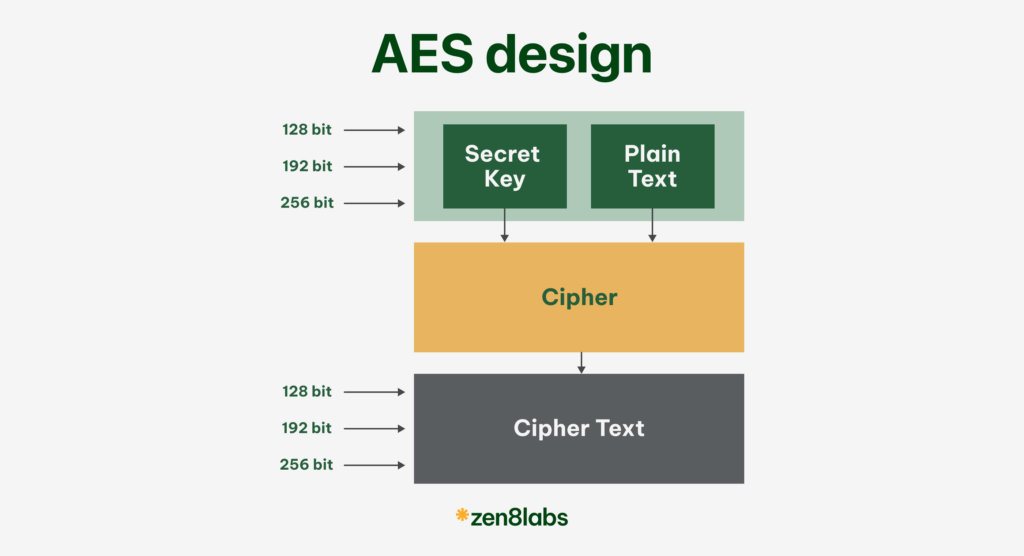
Because of its efficiency and security, the symmetric encryption technique known as AES (Advanced Encryption Standard) is frequently used in many different applications. The term “symmetric encryption” means encryption and decryption using the same key. Different key lengths are supported by AES, with AES-256 being one of the more secure alternatives because of its 256-bit key length. AES runs on set block sizes.
Using an initialization vector (IV) that ensures that identical plaintext blocks return different ciphertext blocks, AES-CBC (Cipher Block Chaining) is an AES mode of operation that improves security. Every encryption process requires a different and unpredictable IV.
We will focus on AES-CBC-256, which combines the extra protection offered by the CBC mode with the strong security of AES.
Setting up the script
Create a shell script file named start.sh with the following content::
```bash
#!/bin/bash
echo "Hello world"
```
Give executable permissions to the script:
```bash
chmod +x start.sh
```
You can then run the script:
```bash
./start.sh
```
The output will show like this:
```
Hello world
```
Implementing the functions
Installing OpenSSL Library
To implement AES encryption and decryption functions, we’ll leverage OpenSSL.
Installation Steps:
For macOS (using HomeBrew):
```
brew install openssl
```
For Ubuntu (using apt):
```
apt-get install libssl-dev
```
Implementing AES encrypt, decrypt & generate key functions
Now, let’s integrate OpenSSL to handle AES encryption and decryption within our shell script.
Here’s how you can incorporate OpenSSL to encrypt and decrypt using AES-256-CBC:
Encrypt function
```
#!/bin/bash
# Function to encrypt with AES using OpenSSL
encrypt_aes() {
read -p "Enter the text to encrypt: " text_to_encrypt
read -p s "Enter the AES key (Base64): " base64_encoded_key
echo # for newline after entering password
local key_hex=$(echo "$base64_encoded_key" | base64 -d | xxd -p -u -c 32)
# Prompt for IV input
read -p "Enter the IV in hexadecimal (leave empty for random IV): " iv_hex_input
if [ -z "$iv_hex_input" ]; then
iv_hex=$(openssl rand -hex 16) # Generate random IV for AES-256-CBC
else
iv_hex=$iv_hex_input
fi
# Encrypt using AES-256-CBC with specified IV
local encrypted_text=$(echo -n "$text_to_encrypt" | openssl enc -aes-256-cbc -iv "$iv_hex" -e -a -K "$key_hex")
echo "AES Encrypted Text: $encrypted_text"
}
```
Decrypt function
```
decrypt_aes() {
read -p "Enter the text to decrypt (Base64): " encrypted_text
read -p -s "Enter the AES key (Base64): " base64_encoded_key
echo # for newline after entering password
local key_hex=$(echo "$base64_encoded_key" | base64 -d | xxd -p -u -c 32)
# Prompt for IV input
read -p "Enter the IV in hexadecimal: " iv_hex
echo "iv_hex: $iv_hex"
echo "key_hex: $key_hex"
# Decrypt using AES-256-CBC with specified IV
decrypted_text=$(echo "$encrypted_text" | openssl enc -aes-256-cbc -iv "$iv_hex" -d -a -K "$key_hex")
if [ $? -eq 0 ]; then
echo "AES Decrypted Text: $decrypted_text"
else
echo "Decryption failed. Check your input and try again."
fi
}
```
Using the script
We have four options in our script:
- Encrypt with AES (Base64 key)
- Decrypt with AES (Base64 key)
- Generate AES key
- Generate IV
Here’s how you can use each option step-by-step:
Generate AES key
This will create a new AES key for encryption and decryption.
- Run the script and select option 3.
- The script will output a Base64 encoded AES key.
- Save this key securely as it is required for both encryption and decryption.
Generate IV
This will create a new initialization vector (IV) for encryption.
- Run the script and select option 4.
- The script will output a hexadecimal IV.
- Save this IV securely as it is required for both encryption and decryption
Encrypt text
This will encrypt the text you provide using the generated AES key and IV.
- Run the script and select option 1.
- Enter the text you want to encrypt when prompted.
- Enter the previously generated AES key when prompted.
- Enter the previously generated IV when prompted (or leave it blank to generate a new IV).
Decrypt text
This will decrypt the encrypted text back to the original text using the same AES key and IV.
- Run the script and select option 2.
- Enter the Base64 encoded encrypted text when prompted.
- Enter the previously generated AES key when prompted.
- Enter the previously generated IV when prompted.
By following these steps, you can successfully encrypt and decrypt text using AES-256-CBC with your generated keys and IVs. This script simplifies the process and ensures that your data is securely transmitted over your APIs.
Full script for reference
```
#!/bin/bash
# Function to encrypt with AES using OpenSSL
encrypt_aes() {
read -p "Enter the text to encrypt: " text_to_encrypt
read -p "Enter the AES key (Base64): " base64_encoded_key
echo # for newline after entering password
local key_hex=$(echo "$base64_encoded_key" | base64 -d | xxd -p -u -c 32)
# Prompt for IV input
read -p "Enter the IV in hexadecimal (leave empty for random IV): " iv_hex_input
if [ -z "$iv_hex_input" ]; then
iv_hex=$(openssl rand -hex 16) # Generate random IV for AES-256-CBC
else
iv_hex=$iv_hex_input
fi
# Encrypt using AES-256-CBC with specified IV
local encrypted_text=$(echo -n "$text_to_encrypt" | openssl enc -aes-256-cbc -iv "$iv_hex" -e -a -K "$key_hex")
echo "AES Encrypted Text: $encrypted_text"
}
# Function to decrypt with AES using OpenSSL
decrypt_aes() {
read -p "Enter the text to decrypt (Base64): " encrypted_text
read -p "Enter the AES key (Base64): " base64_encoded_key
echo # for newline after entering password
local key_hex=$(echo "$base64_encoded_key" | base64 -d | xxd -p -u -c 32)
# Prompt for IV input
read -p "Enter the IV in hexadecimal: " iv_hex
echo "iv_hex: $iv_hex"
echo "key_hex: $key_hex"
# Decrypt using AES-256-CBC with specified IV
decrypted_text=$(echo "$encrypted_text" | openssl enc -aes-256-cbc -iv "$iv_hex" -d -a -K "$key_hex")
if [ $? -eq 0 ]; then
echo "AES Decrypted Text: $decrypted_text"
else
echo "Decryption failed. Check your input and try again."
fi
}
# Function to generate AES key
generate_aes_key() {
aes_key=$(openssl rand -hex 32) # Generate random 32-byte AES key in hexadecimal format
aes_key_base64=$(echo -n "$aes_key" | xxd -r -p | base64)
echo "Generated AES Key (Base64): $aes_key_base64"
}
# Function to generate IV
generate_iv() {
iv_hex=$(openssl rand -hex 16) # Generate random IV for AES-256-CBC
echo "Generated IV: $iv_hex"
}
# Function to display menu
show_menu() {
echo "Choose a function:"
echo "1) Encrypt with AES (Base64 key)"
echo "2) Decrypt with AES (Base64 key)"
echo "3) Generate AES key"
echo "4) Generate IV"
echo -n "Enter your choice [1-4]: "
}
# Main script execution
show_menu
read choice
case $choice in
1) encrypt_aes ;;
2) decrypt_aes ;;
3) generate_aes_key ;;
4) generate_iv ;;
*) echo "Invalid choice, please try again." ;;
esac
```
Conclusion
Using this Bash script, you can efficiently manage AES encryption and decryption for your mobile applications. By automating key and IV generation, as well as providing straightforward encryption and decryption functions, this script simplifies the process of securing your data.
Hope this helps you to create your own script that assists in your work. Feel free to customize it further to suit your specific needs!
Quan Nguyen, Solutions Architect